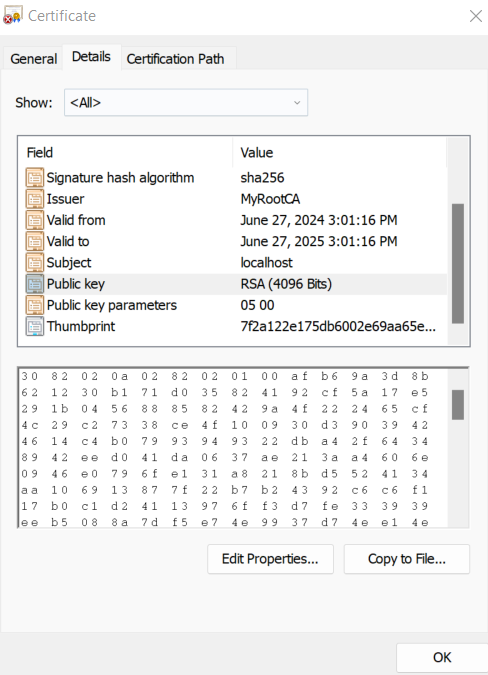
In the world of cybersecurity, public keys play a vital role in securing communication and verifying digital signatures. Often, these public keys reside within certificates stored in the Windows Certificate Store. Extracting these keys programmatically can be a valuable tool for various applications, such as certificate validation or key management automation. How to programmatically extract a public key using Win32 for a certificate stored in a certificate store? This blog delves into the process of achieving this using the Win32 API in C/C++.
- Win32 API for certificate management
- Extracting public keys from certificates in C/C++
- Programmatic access to Windows Certificate Store
Delving into Win32 API for Certificate Operations
Let’s know how to programmatically extract a public key using Win32 for a certificate stored in a certificate store? The Win32 API offers a suite of functions for interacting with the Windows Certificate Store. Here’s a breakdown of the key functions involved in extracting public keys:
- CertOpenStore: This function establishes a handle to a specific certificate store. You’ll need to specify the store location (e.g., Current User’s certificate store) and the store type (e.g., CERT_STORE_PROV_SYSTEM).
- CertFindCertificateInStore: Once you have a store handle, this function helps locate the desired certificate within the store. You can search based on various criteria, such as subject name, serial number, or issuer information.
- CertCreateCertificateContext: With the certificate pointer obtained from
CertFindCertificateInStore
, this function creates a certificate context object that encapsulates all the certificate details. - CryptDecodeObjectEx: Here comes the key extraction part. This function decodes the Subject Public Key Info (SPKI) embedded within the certificate context. You’ll need to specify the format (e.g., X509_ASN_ENCODING) and the desired key structure (e.g., for RSA keys, RSA_CSP_PUBLICKEYBLOB).
- Accessing the Public Key Data: After successful decoding, the
CryptDecodeObjectEx
function populates a structure containing the public key information. This structure typically includes fields for the key algorithm identifier, key size, and the actual public key data itself.
Putting it all Together: Code Example
Here’s a simplified code snippet demonstrating the core functionalities:
C++
#include <windows.h>
#include <wincrypt.h>
// Function to extract the public key from a certificate
PCCERT_CONTEXT ExtractPublicKey(HCERTSTORE hStore, LPCSTR subjectName) {
// Find the certificate by subject name
PCCERT_CONTEXT pCertContext = CertFindCertificateInStore(
hStore, CERT_FIND_SUBJECT_STR, 0, NULL, subjectName, CERT_FIND_ANY);
if (!pCertContext) {
// Handle certificate not found error
return NULL;
}
// Create certificate context
pCertContext = CertCreateCertificateContext(X509_ASN_ENCODING,
pCertContext->pbCertEncoded,
pCertContext->cbCertEncoded);
if (!pCertContext) {
// Handle certificate context creation error
CertFreeCertificateContext(pCertContext);
return NULL;
}
// Allocate memory for the public key structure (e.g., RSA_CSP_PUBLICKEYBLOB)
DWORD keyBlobSize = 0;
CryptDecodeObjectEx(X509_ASN_ENCODING, CERT_PUBLICKEY_INFO,
pCertContext->pCertInfo->SubjectPublicKeyInfo.pbData,
pCertContext->pCertInfo->SubjectPublicKeyInfo.cbData,
CRYPT_DECODE_ALLOC_FLAG, NULL, &keyBlobSize, NULL, NULL);
BYTE* pPublicKeyBlob = new BYTE[keyBlobSize];
// Extract the public key
if (CryptDecodeObjectEx(X509_ASN_ENCODING, CERT_PUBLICKEY_INFO,
pCertContext->pCertInfo->SubjectPublicKeyInfo.pbData,
pCertContext->pCertInfo->SubjectPublicKeyInfo.cbData,
CRYPT_DECODE_ALLOC_FLAG, NULL, &keyBlobSize, pPublicKeyBlob, NULL)) {
// Use the extracted public key data (pPublicKeyBlob)
// …
// Free allocated memory
delete[] pPublicKeyBlob;
} else {
// Handle public key
YOU MAY BE INTERESTED IN:
SAP vs. BLM: Understanding Two Completely Different Acronyms
SAP HANA Cloud Tools: Empowering Development and Administration in the