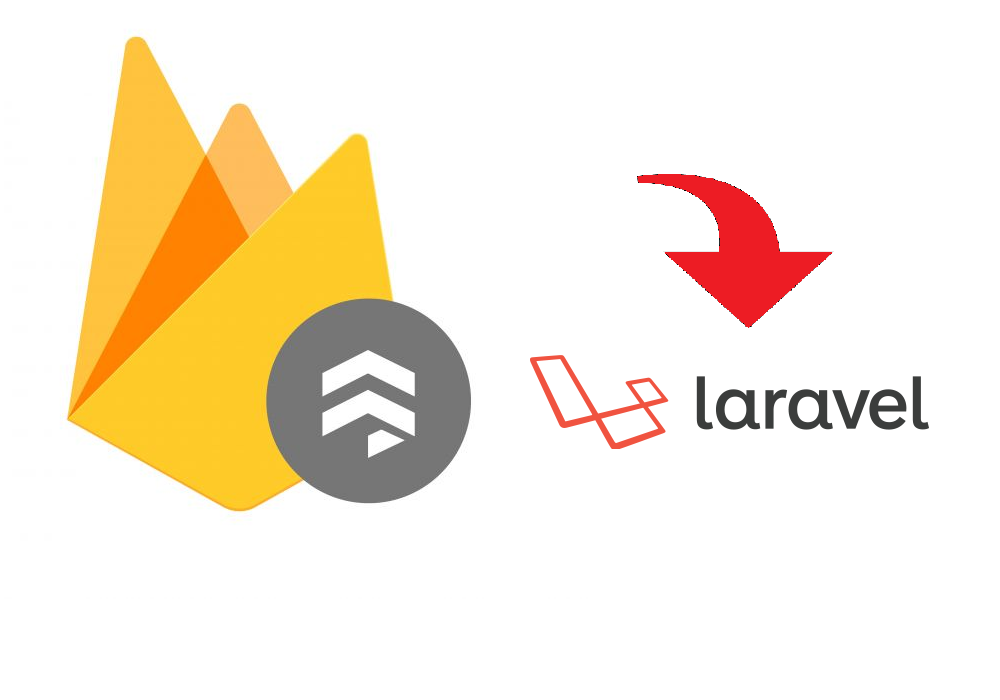
Introduction
Firebase Firestore, a NoSQL database from Google, offers a powerful and scalable solution for web and mobile app development. Its flexible data structure and real-time updates make it a popular choice for dynamic applications. However, when dealing with large collections containing thousands of documents, fetching all data at once becomes inefficient and impractical. This blog post dives deep into effective strategies for retrieving more than 5,000 documents from Firestore using Laravel, a popular PHP framework. and answer your question, How to fetch more than 5000 documents from firestore using Laravel?
Understanding Firestore Limitations
Firestore enforces a document size limit and discourages fetching entire collections due to potential performance issues and network latency. Retrieving a massive dataset in one go can overwhelm your application and lead to slow loading times.
Approaches for Efficient Data Retrieval
Here are several effective methods to fetch large datasets from Firestore in your Laravel application:
- Cursor Pagination:
- Firestore provides built-in cursor functionality for paginating results. This approach utilizes
limit
andstartAfter
orendBefore
clauses to retrieve a defined number of documents (page size) at a time. - In your Laravel controller, leverage the
firestore
facade provided by the Firebase PHP SDK. You can construct queries with the desired page size and utilize thegetDocuments()
method with appropriate cursor parameters. - This method is efficient as it retrieves only the requested data and avoids loading the entire collection.
- Firestore provides built-in cursor functionality for paginating results. This approach utilizes
- Query by Field:
- If your data retrieval has specific criteria, leverage Firestore queries to filter documents based on specific fields. This significantly reduces the amount of data fetched, improving performance.
- Laravel’s
where
methods offered by thefirestore
facade seamlessly integrate with Firestore queries. You can define conditions on document fields to narrow down the result set.
- Cloud Functions & Background Jobs:
- For particularly large datasets, consider offloading the data retrieval process to a background job or Cloud Function. This prevents blocking the main application thread and ensures a responsive user experience.
- Laravel offers robust queueing mechanisms like Laravel Horizon or a dedicated queueing system like Beanstalkd. You can create a background job that fetches data from Firestore and stores it in a cache or database for later use.
- Firestore Offline Persistence:
- Firestore offers offline persistence capabilities, allowing you to cache frequently accessed data locally on the client-side (mobile app or browser). This enables retrieval of essential documents even when offline, enhancing user experience.
- The Firebase JavaScript SDK provides methods for enabling offline persistence and managing cached data.
Optimizing Performance
- Indexing: Properly indexing relevant fields in your Firestore collections significantly improves query performance, especially when using query by field approach.
- Data Structure: Structure your Firestore data efficiently to minimize the need for complex queries and data manipulation on the client-side.
Code Example (Cursor Pagination):
PHP
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Kreait\Firebase\Firestore;
class DocumentController extends Controller
{
public function getDocuments(Request $request)
{
$pageSize = 10; // Define your desired page size
$pageToken = $request->get('pageToken'); // Get pagination token from request
$firestore = app('firebase.firestore');
$collection = $firestore->database()->collection('documents');
$query = $collection->limit($pageSize);
if ($pageToken) {
$query = $query->startAfter($pageToken);
}
$documents = $query->getDocuments();
// Process and return retrieved documents and next page token (if applicable)
return response()->json([
'documents' => $documents->toArray(),
'nextPageToken' => $documents->getNextPageToken()
]);
}
}
Use code with caution.content_copy
Conclusion
By adopting these strategies, you can efficiently retrieve large datasets from Firestore within your Laravel application. Remember to choose the approach that best aligns with your specific data access patterns and performance requirements. By implementing proper indexing, optimizing data structure, and utilizing background jobs when necessary, you can ensure a smooth user experience even when dealing with significant data volumes in Firestore.
YOU MAY LIKE THIS