In the realm of C++ programming, efficiently managing data structures is paramount. Often, we encounter scenarios where we need to extract meaningful information from a string and organize it in a structured manner. This is where the power of std::map
comes into play. But how do we bridge the gap between a raw string and a well-defined map? This blog post delves into the nitty-gritty of converting a C++ std::string
to an std::map
, empowering you to tackle this task with confidence.
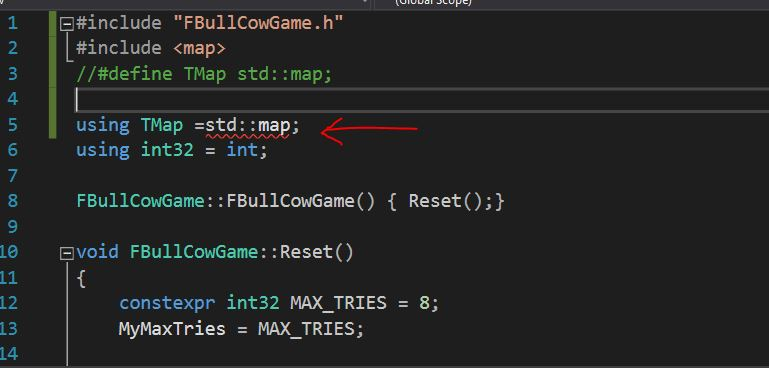
Understanding the Why: When Conversion Reigns Supreme
There are several compelling reasons why converting a std::string
to a std::map
might be necessary in your C++ std code:
- Data Parsing: Imagine a string holding comma-separated key-value pairs. Converting it to a
std::map
allows you to efficiently access each key-value combination for further processing. - Configuration Files: Many applications rely on configuration files stored as strings. By converting these strings to maps, you can easily retrieve configuration parameters based on their corresponding keys.
- Structured Data Extraction: When working with data retrieved from APIs or external sources, it might be delivered as a string. Converting it to a map provides a structured format for manipulation and analysis.
Unveiling the Approaches: Techniques for String-to-Map Conversion
While there’s no built-in function for direct conversion, here are two effective techniques to transform your std::string
into a std::map
:
1. Leveraging String Streams and Iterators:
- Step 1: Stream Establishment: Create an
std::istringstream
object from your input string. This stream acts as a character buffer, allowing you to treat the string as a sequence of characters. - Step 2: Delimitation Dance: Employ a delimiter (often a comma ‘,’) to separate key-value pairs within the string. Use the
getline
function of the stream to extract each delimited token. - Step 3: The Parsing Powerhouse: Split each token further using another delimiter (often a colon ‘:’) to isolate the key and value.
- Step 4: Map Marvels: Employ the
std::map
constructor that takes an iterator range. This allows you to iterate through the extracted key-value pairs and insert them into the map.
Code Example:
C++ std
#include <iostream>
#include <sstream>
#include <map>
#include <string>
std::map<std::string, std::string> stringToMap(const std::string& str) {
std::map<std::string, std::string> result;
std::istringstream iss(str);
std::string key, value;
while (std::getline(iss, key, ':') && std::getline(iss, value)) {
result[key] = value;
}
return result;
}
int main() {
std::string inputString = "name:Alice,age:30,city:New York";
std::map<std::string, std::string> dataMap = stringToMap(inputString);
for (const auto& [key, value] : dataMap) {
std::cout << key << ": " << value << std::endl;
}
return 0;
}
Use code with caution.content_copy
2. Regular Expressions: A Powerful Ally
- Step 1: Regular Expression Arsenal: Craft a regular expression that captures key-value pairs within the string. This expression typically includes delimiters and captures key and value groups.
- Step 2: Regex Magic: Employ the
std::regex_search
function to identify matches of the regular expression within the string. - Step 3: Map Mastery: Iterate through the matched key-value pairs and insert them into the
std::map
.
Code Example (using C++11 features):
C++
#include <iostream>
#include <regex>
#include <map>
#include <string>
std::map<std::string, std::string> stringToMap(const std::string& str) {
std::map<std::string, std::string> result;
std::regex pattern(R"(\{([^"]+)\s*,\s*([^"]+)\})"); // Captures keys and values
std::smatch matches;
std::string::const_iterator searchStart(str.cbegin());
while (std::regex_search(searchStart, str.cend(), matches, pattern)) {
result[matches
YOU MAY LIKE THIS
Mastering Customer 360 in Salesforce
Cracking the Code: SAP Functional Analyst Salary at Deloitte India