Build a Kubernetes client with Go SDK for AWS EKS with automatic token refresh
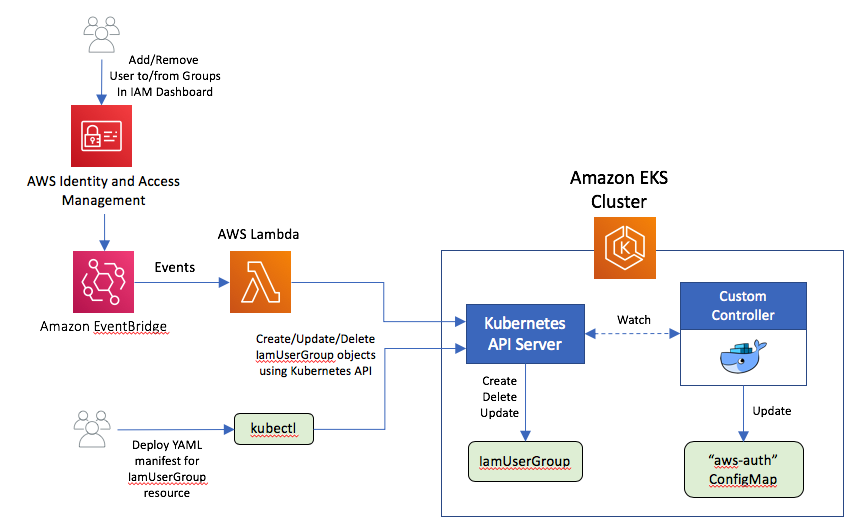
Introduction
build a Kubernetes client with Go SDK for AWS EKS with automatic token refresh? Integrating your Go application with an Amazon Elastic Kubernetes Service (EKS) cluster requires interacting with the Kubernetes API server. The Go client library for Kubernetes (https://github.com/kubernetes/client-go) provides a robust solution for this purpose. However, for out-of-cluster applications, managing token expiration and refresh becomes a crucial aspect. This blog delves into crafting a Kubernetes client in Go that seamlessly interacts with your AWS EKS cluster, including automatic token refresh for uninterrupted communication.
Understanding the Challenge
When interacting with a Kubernetes API server from outside the cluster (out-of-cluster), standard kubeconfig files are not suitable. These files are primarily designed for in-cluster deployments where service account tokens are automatically mounted and managed. For out-of-cluster scenarios, we need to obtain tokens from the AWS STS service and implement a mechanism to refresh them before they expire.
Building a Kubernetes client with Go SDK for AWS EKS with automatic token refresh
Here’s a breakdown of the steps involved:
- Retrieving Cluster Information:
- Leverage the AWS SDK for Go (https://docs.aws.amazon.com/sdk-for-go/api/) to interact with the EKS service.
- Use the
DescribeCluster
API call to fetch details like the cluster endpoint and cluster CA certificate.
- Obtaining AWS Credentials:
- Implement logic to retrieve your AWS credentials securely. This could involve environment variables, IAM roles, or a credential provider.
- Generating Tokens with AWS STS:
- Utilize the
GetSessionToken
API call from the AWS STS service to obtain temporary credentials containing an access token. These tokens typically have a validity period of one hour.
- Utilize the
- Creating the Kubernetes Client Configuration:
- Construct a
rest.Config
object using the retrieved cluster endpoint, CA certificate, and access token.
- Construct a
- Implementing Automatic Token Refresh:
- Create a custom
RoundTripper
type that intercepts outgoing requests to the Kubernetes API server. - Within the
RoundTripper
, check the token expiration time. - If the token is nearing expiry (e.g., within a defined threshold), use the AWS STS service again to fetch fresh credentials and update the access token in the
rest.Config
object. - Wrap the original HTTP client with your custom
RoundTripper
to ensure automatic token refresh before each request.
- Create a custom
- Building the Kubernetes Clientset:
- Employ the
kubernetes.NewForConfig
function to construct a Kubernetes clientset using the configuredrest.Config
object. This clientset provides access to various Kubernetes API resources like deployments, pods, and services.
- Employ the
Code Example (Illustrative Purposes Only):
Go
package main
import (
"context"
"fmt"
"time"
"github.com/aws/aws-sdk-go/aws"
"github.com/aws/aws-sdk-go/aws/session"
"github.com/aws/aws-sdk-go/service/eks"
"github.com/aws/aws-sdk-go/service/sts"
"github.com/go-restful/restful"
"k8s.io/client-go/kubernetes"
)
type tokenRefresherRoundTripper struct {
restful.RoundTripper
token string
expiry time.Time
threshold time.Duration
}
func (t *tokenRefresherRoundTripper) RoundTrip(req *restful.Request) (*restful.Response, error) {
if time.Now().After(t.expiry.Add(-t.threshold)) {
// Refresh token logic using AWS STS
// Update token and expiry in rest.Config
}
return t.RoundTripper.RoundTrip(req)
}
func main() {
// Get cluster information and AWS credentials (omitted for brevity)
// Create STS client
stsSvc := sts.New(session.New())
// Get initial token
tokenResult, err := stsSvc.GetSessionToken(&sts.GetSessionTokenInput{})
if err != nil {
// Handle error
}
// Configure rest.Config with cluster details and initial token
config := &rest.Config{
// ...
Token: aws.String(*tokenResult.Credentials.SessionToken),
}
// Create custom RoundTripper with refresh logic
rt := &tokenRefresherRoundTripper{
RoundTripper: restful.NewFakeRoundTripper(),
token: *tokenResult.Credentials.SessionToken,
YOU MAY BE INTERESTED IN
How to Download CUET 2024 Answer Key: A Step-by-Step Guide
Best SAP ERP Implementers and Consultants for 2024
Difference between ECC and S4 HANA