In the realm of object-oriented programming (OOP), Java’s abstract classes serve as a cornerstone for code reusability, flexibility, and promoting well-defined inheritance hierarchies. This blog post delves into the intricate details of Abstract Classes in Java, explores their practical applications, and sheds light on best practices for their effective utilization.
Understanding Abstract Classes in Java: The Core Concept
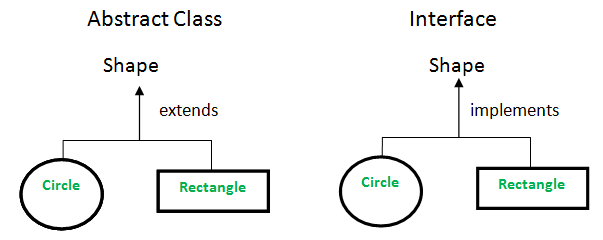
An abstract Classes in Java acts as a blueprint for subclasses, outlining the overall structure and defining shared functionalities. Unlike concrete classes that can be instantiated to create objects, abstract classes themselves cannot be directly instantiated. Their primary purpose lies in providing a foundation for subclasses to inherit and extend upon.
Syntactic Breakdown: Crafting Abstract Classes
The abstract
keyword is the heart and soul of abstract class declaration in Java. Here’s a breakdown of the syntax:
Java
abstract class AbstractClassName {
// Abstract methods (without implementation)
public abstract void abstractMethodName();
// Concrete methods (with implementation)
public void concreteMethodName() {
// Method body
}
// Fields (can be abstract or non-abstract)
public abstract int abstractField;
public int nonAbstractField;
}
Use code with caution.content_copy
Let’s dissect the key elements:
abstract
keyword: This keyword declares the class as abstract, preventing direct object creation.- Abstract methods: These methods lack an implementation (method body) and are declared using the
abstract
keyword. Subclasses must override these methods to provide specific functionality. - Concrete methods: These methods, on the other hand, possess a complete implementation within the abstract class itself and can be invoked directly from subclasses.
- Fields: Abstract classes can have both abstract and non-abstract fields. Abstract fields, similar to abstract methods, require subclasses to provide their definitions.
The Power of Inheritance: Subclasses Take the Stage
The true strength of abstract classes lies in their synergy with inheritance. Subclasses extend abstract classes, inheriting their non-abstract methods and fields, and overriding abstract methods to provide concrete implementations. This mechanism fosters code reuse and promotes a well-organized inheritance structure.
Illustrative Example: Unveiling the Power of Abstraction
Consider a scenario where you want to design a program for various shapes, each with its own area calculation logic. Here’s how abstract classes can streamline the process:
Java
abstract class Shape {
public abstract double calculateArea();
public void printArea() {
System.out.println("Area: " + calculateArea());
}
}
class Square extends Shape {
private double sideLength;
public Square(double sideLength) {
this.sideLength = sideLength;
}
@Override
public double calculateArea() {
return sideLength * sideLength;
}
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double calculateArea() {
return Math.PI * radius * radius;
}
}
public class Main {
public static void main(String[] args) {
Square square = new Square(5);
Circle circle = new Circle(3);
square.printArea(); // Output: Area: 25.0
circle.printArea(); // Output: Area: 28.274333882308138
}
}
Use code with caution.content_copy
In this example, the Shape
class is abstract, defining the calculateArea()
method without an implementation. The Square
and Circle
subclasses extend Shape
, override the abstract method to provide their specific area calculation logic, and leverage the inherited printArea()
method. This demonstrates code reusability and promotes a clean separation of concerns.
Best Practices for Effective Abstract Class Usage
- Clear Abstraction: Clearly define the core functionalities and responsibilities within the abstract class, ensuring a well-defined contract for subclasses.
- Minimal Abstract Methods: Aim to keep the number of abstract methods minimal, promoting concrete implementations within subclasses whenever possible.
- Final Abstract Methods: If a specific behavior shouldn’t be overridden by subclasses, declare the abstract method as
final
to prevent modification. - Template Method Pattern: Utilize the template method pattern to define a skeletal algorithm within the abstract class, allowing subclasses to customize specific steps.
By adhering to these best practices, you can leverage abstract classes effectively to create robust and maintainable object-oriented applications in Java.
Conclusion
Abstract classes in Java offer a powerful mechanism for achieving code reusability, promoting flexibility through inheritance, and fostering well-info.
Sources
- github.com/MichalKowalczewski/Byd13
- github.com/Rafayel02/com.ACA.JAVA
- github.com/AdamWaszak102/java_programs
YOU MAY BE INTERESTED IN
Configuring US Benefits with SAP: SAP PRESS Essentials 40