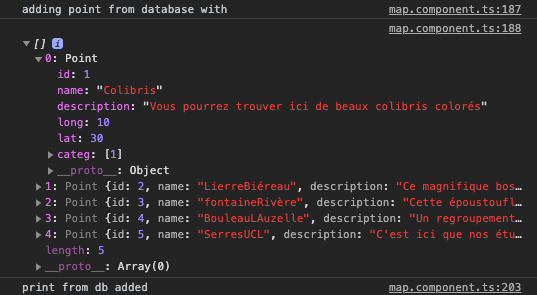
Mastering Object Creation and Iteration in TypeScript: From Arrays to Powerful Data Structures
In the dynamic world of TypeScript development, efficiently manipulating data is crucial. Often, you’ll encounter scenarios where you need to transform information from arrays into structured objects for further processing. This blog dives deep into Object Creation and Iteration in TypeScript, crafting objects from arrays in TypeScript, along with exploring various techniques to iterate over their keys and unlock the full potential of your data.
Understanding the Why: When Arrays Become Objects
Arrays, while excellent for storing ordered collections of elements, lack the organization offered by objects. Objects, on the other hand, provide a key-value pair structure, making data retrieval and manipulation significantly faster. Imagine an array containing user information – names, emails, and IDs. While iterating through the array to find a specific user by ID would be cumbersome, converting it to an object with IDs as keys allows for instant lookup using bracket notation.
Crafting Objects from Arrays: Unveiling the Methods
TypeScript empowers you with a robust toolkit to create objects from arrays. Let’s explore the most common methods:
- Object.fromEntries(): This built-in function streamlines object creation from an iterable of key-value pairs. It accepts an array of arrays, where each inner array represents a key-value pair. Here’s an example:
TypeScript
const userDataArray = [["name", "Alice"], ["email", "alice@example.com"], ["id", 123]];
const userObject = Object.fromEntries(userDataArray);
console.log(userObject); // { name: "Alice", email: "alice@example.com", id: 123 }
Use code with caution.content_copy
- Reduce Method: This versatile method iterates over the array, accumulating a single object. It takes an accumulator function and an initial value as arguments. Inside the accumulator, you can construct the object by adding key-value pairs using the spread operator (
...
).
TypeScript
const productDataArray = [
{ id: 1, name: "Shirt", price: 25 },
{ id: 2, name: "Hat", price: 15 },
];
const productObject = productDataArray.reduce((acc, product) => {
return { ...acc, [product.id]: product };
}, {});
console.log(productObject); // { 1: { id: 1, name: "Shirt", price: 25 }, 2: { id: 2, name: "Hat", price: 15 } }
Use code with caution.content_copy
Conquering Object Keys: Iteration Techniques
Once you have your object crafted, it’s time to unlock its potential. Here are the prominent ways to iterate over object keys in TypeScript:
- for…in Loop: This loop is specifically designed to iterate over enumerable properties of an object. It provides the key name during each iteration, allowing you to access the corresponding value.
TypeScript
const personObject = { name: "Bob", age: 30, city: "New York" };
for (const key in personObject) {
console.log(`${key}: ${personObject[key]}`);
}
Use code with caution.content_copy
- Object.keys() Method: This method returns an array containing all the enumerable keys of an object. You can then use a traditional
for
loop or array iteration methods likeforEach
ormap
to process each key.
TypeScript
const carObject = { make: "Ford", model: "Mustang", year: 2023 };
Object.keys(carObject).forEach(key => {
console.log(key, carObject[key]);
});
Use code with caution.content_copy
Choosing the Right Method: A Strategic Approach
When selecting a method for object creation or iteration, consider these factors:
- Data Structure of the Array: If your array already contains key-value pairs,
Object.fromEntries()
is a clear winner. - Performance Requirements: For large datasets, the
reduce
method might be slightly more performant due to its single loop iteration. - Readability and Maintainability: Both
Object.fromEntries()
and thereduce
method offer clean syntax for complex object creation logic. - Iteration Needs: If you only need the keys,
Object.keys()
is efficient. For accessing both keys and values, loops are necessary.
Beyond the Basics: Advanced Object Manipulation
TypeScript empowers you with advanced techniques to manipulate objects further:
- Destructuring: Extract specific key-value pairs from objects during creation or iteration.
- Spread Operator: Efficiently merge objects and create copies with modifications.
- Type Annotations: Enforce type safety for object properties, enhancing code reliability.
YOU MAY BE INTERESTED
Chat GPT: The Power of Artificial Intelligence in Conversational Interfaces
A Comprehensive Guide to SAP S4hana Upgrade steps
Inter Supply Results 2024: All You Need to Know